Introduction
Adding QR code generation and download features to a React.js app makes sharing information easy. QR codes can store data like website links, text, and images. They were first used for product labeling and inventory tracking but are now common in daily life. You can find them in UPI payments, WhatsApp contact sharing, and Play Store app links.
In this blog, we’ll show you how to generate and download QR codes in a React.js app. This will let users create, save, share, or print their own QR codes with ease.
Prerequisites
- Node.js and npm
- React
- MUI
- MUI Icons
- Qrcode
- react-color
Use Cases of Custom QR Codes in Your React App
- Product Information : Generate QR codes that link to product details like pricing and reviews.
- Event Ticketing : Use QR codes for concert, flight, or conference tickets, allowing quick access for event entry.
- Contact Information : Share contact details easily through QR codes.
- App Downloads : Direct users to your app’s store page via QR codes for simple installation.
- Wi-Fi Sharing : Share Wi-Fi credentials with a scannable QR code for easy connection.
- Payment Links : Generate QR codes for payment links via UPI or PayPal.
- Dynamic QR Codes for Marketing : Use QR codes to track marketing campaigns, offering promotions or discounts.
We’ll show you how to generate and customize QR codes in React.js to enhance your app’s functionality.
Start the React App
If you don’t have a React project yet, you can make one like this:
//Create react app using the following command
npx create-react-app qrcode_generator
//Install the required library
npm i @mui/material @emotion/react @emotion/styled @mui/icons-material
npm i qrcode
npm i react-color
About qrcode Library
The qrcode library is a versatile tool for generating QR codes in React. It supports customization, allowing you to create scannable codes for URLs, text, and more. With options for data types, sizes, and error correction, it simplifies adding QR functionality to your app.
In our React project, App.js serves as the main entry point. It manages state, processes user actions, and renders core components. Below is the code for App.js:
//Necessary Imports
function App() {
const [qrData, setQrData] = useState({ link: "", fgColor: "#000000", bgColor: "#ffffff" });
const handleQRCodeGenerate = (link, fgColor, bgColor) => {
setQrData({ link, fgColor, bgColor });
};
return (
);
}
export default App;
In this code, the App.js component manages the QR code generation functionality by using React state and passing data between two child components.
Functionality
- State Management :
- The GenerateQR component receives the latest qrData (link, foreground color, and background color) from the state. It is responsible for generating and displaying the QR code based on these values.
- The main idea is that QRConfiguration captures user input, and GenerateQR updates the QR code dynamically as the state changes.
- handleQRCodeGenerate Function :
- This function is passed down as a prop to the QRConfiguration component.
- When the QRConfiguration component triggers the QR code generation, this function updates the qrData state with the new values for link, fgColor, and bgColor.
- Rendering the QR Code :
- The GenerateQR component receives the latest qrData (link, foreground color, and background color) from the state. It generates and displays the QR code based on these values.
The main function is to capture user input in QRConfiguration and update the QR code in GenerateQR dynamically as the state changes
//Necessary Imports
function QRConfiguration({ onQRCodeGenerate }) {
const [link, setLink] = useState("");
const [fgColor, setFgColor] = useState("#000000");
const [bgColor, setBgColor] = useState("#ffffff");
const [showColorPicker, setShowColorPicker] = useState(false);
const handleCreateQRCode = () => {
onQRCodeGenerate(link, fgColor, bgColor);
};
const handleColorChange = (color, type) => {
if (type === "fgColor") {
setFgColor(color.hex);
} else if (type === "bgColor") {
setBgColor(color.hex);
}
};
return (
Generate Your Custom QR Code
setLink(e.target.value)}
/>
{showColorPicker && (
Pick QR Code Foreground Color:
handleColorChange(color, "fgColor")}
/>
Pick QR Code Background Color:
handleColorChange(color, "bgColor")}
/>
)}
);
}
export default QRConfiguration;
The QRConfiguration component lets users customize their QR code by entering a URL and choosing foreground and background colors. It stores these values in the state. When the “Generate QR Code” button is clicked, it calls onQRCodeGenerate with the selected details. The button stays disabled until a valid URL is entered.
//Necessary Imports
function GenerateQR({ link, fgColor, bgColor }) {
const [isGenerating, setIsGenerating] = useState(true);
const [qrCodeDataUrl, setQrCodeDataUrl] = useState("");
useEffect(() => {
if (link) {
setIsGenerating(true);
QRCode.toDataURL(link, { color: { dark: fgColor, light: bgColor } })
.then((url) => {
setQrCodeDataUrl(url);
setIsGenerating(false);
})
.catch((err) => {
console.error(err);
setIsGenerating(false);
});
}
}, [link, fgColor, bgColor]);
const handleDownload = () => {
if (qrCodeDataUrl) {
const link = document.createElement("a");
link.href = qrCodeDataUrl;
link.download = "qrcode.png";
link.click();
}
};
return (
{isGenerating ? (
) : (
<>
>
)}
);
}
export default GenerateQR;
Output
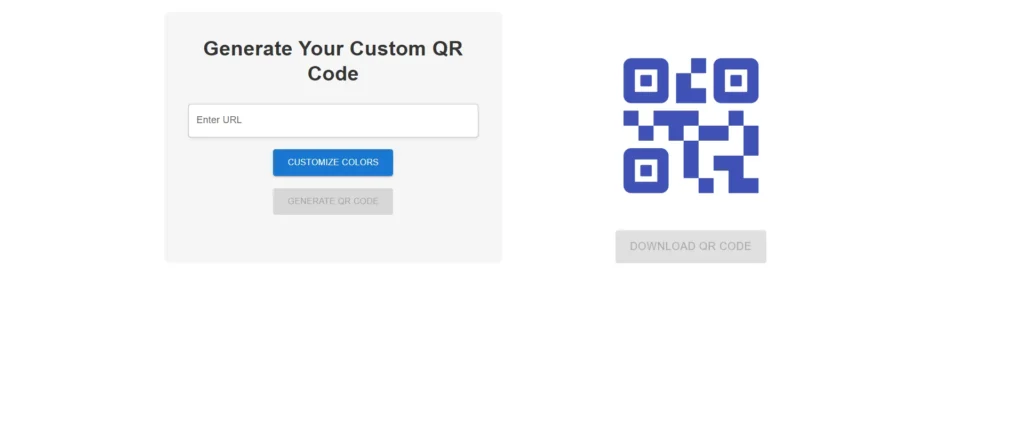
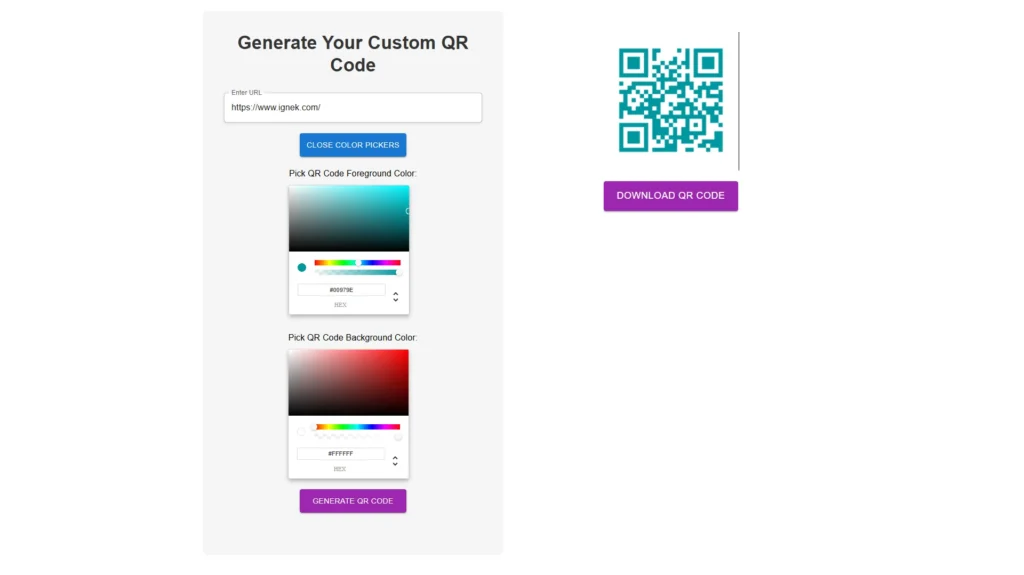
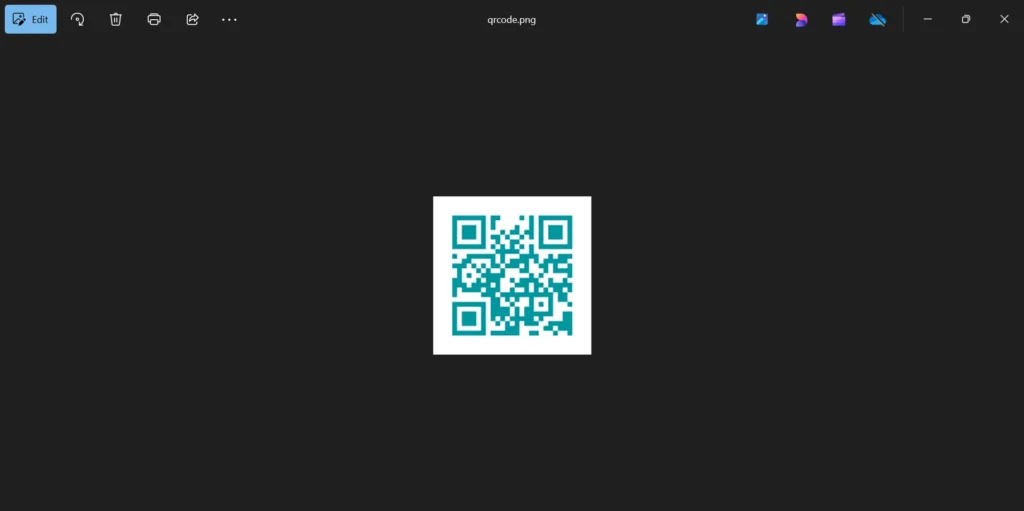
Conclusion
In conclusion, this React app allows users to easily generate custom QR codes by inputting a URL and selecting foreground and background colors. The app efficiently manages state and dynamically updates the QR code based on user preferences.