Introduction
Data visualization is a crucial aspect of modern web applications,enabling users to understand complex datasets through interactive and engaging charts. Visual representation of data is essential for understanding patterns, trends, and insights. Whether you’re developing a dashboard, financial application, or analytics tool, effective data visualization helps users interpret complex information quickly and intuitively.
In React, several libraries are available for charting . But Victory Chart is a powerful and flexible charting library designed specifically for React applications. Victory simplifies data visualization by providing a declarative, component-based API that makes creating stunning and interactive charts easy.
Prerequisites
- Node.js and npm
- React
- Typescript
Why Use Victory?
Victory is a popular and powerful charting library in React. It helps developers create interactive and customizable charts. It also provides many components like VictoryPie, VictoryBar, VictoryChart, and more, which can be easily customized and animated.
Frontend Setup in React
Let’s create a React app using the following command:
//Create a react app using the following command
npx create-react-app victory-chart --template typescript
cd victory-chart
//Install the victory library
npm install victory
//Command for run the React project
npm run dev
Graph with Custom Data
In Victory Charts, you can create a custom chart with your data and specify axis names using VictoryChart, VictoryAxis, and VictoryLine (or other chart components like VictoryBar, VictoryScatter, etc.).
Here’s a basic example of a Victory line chart with custom data and axis labels:
//App.tsx
import ' ./App.css '
import { VictoryAxis , VictoryChart , VictoryLine } from 'victory'
function App() {
const data = [
{ x: "Jan", y: 10 },
{ x: "Feb", y: 30 },
{ x: "Mar", y: 20 },
{ x: "Apr", y: 50 },
{ x: "May", y: 40 },
{ x: "Jun", y: 70 },
];
return (
<>
{/* X-Axis */}
{/* Y-Axis */}
{/* Line Chart */}
>
)
}
export default App
With the above code, you will get the following line chart on your page
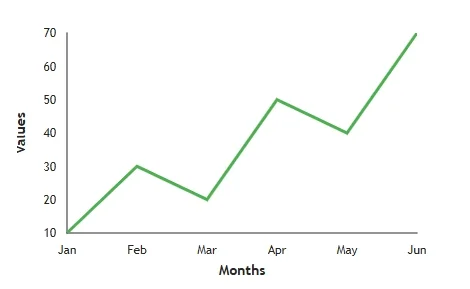
Styling Graph
Victory Charts provide powerful customization options for styling graphs.So you can modify various elements like lines, bars, axes, labels, grid lines, tooltips, and backgrounds to enhance the appearance of your chart.
//App.tsx
import ' ./App.css '
import { VictoryAxis , VictoryBar , VictoryChart } from 'victory'
function App() {
const data = [
{ x: "Jan", y: 10 },
{ x: "Feb", y: 30 },
{ x: "Mar", y: 20 },
{ x: "Apr", y: 50 },
{ x: "May", y: 40 },
{ x: "Jun", y: 70 },
];
return (
{/* X-Axis */}
{/* Y-Axis */}
{/* Line Chart */}
)
}
export default App
With the above code, you will get the following bar chart on your page
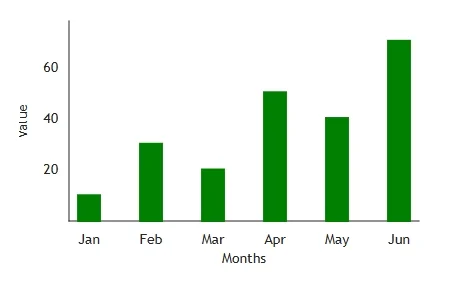
Conclusion
Victory Charts provides a flexible ,powerful ,and easy-to-use library for building data visualizations in React applications. With built-in components like VictoryLine, VictoryBar, and VictoryPie, developers can create a wide range of charts with minimal effort. Victory is ideal for developers who want to create visually appealing and dynamic charts without complex configurations. Whether for simple or advanced visualizations, Victory provides all the necessary tools to create high-quality charts efficiently.